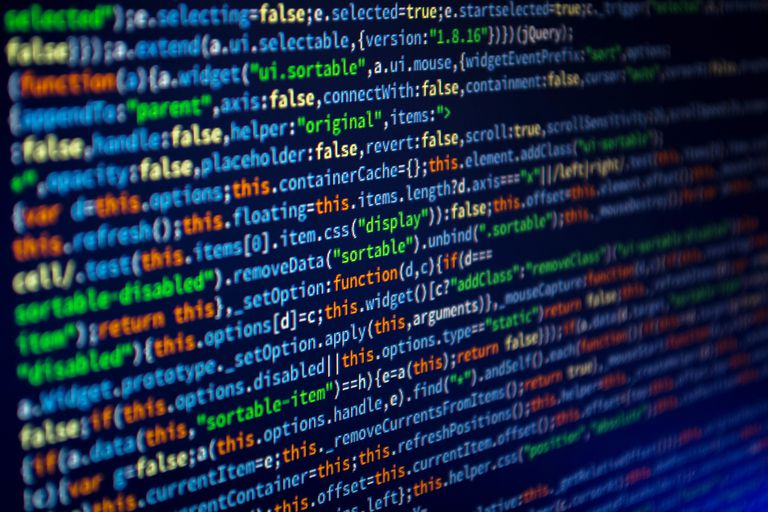
Creating websites that are reactive and responsive used to be considered advanced but responsiveness is a necessity for successful websites and apps. We’ve added media queries, matchMedia
, and a host of other APIs to help developers make responsiveness easier and now we get a new one: ResizeObserver
. With the Resize Observer API, we can watch for resizing on individual elements!
Using ResizeObserver
To listen for resize changes on elements, create a ResizeObserver
instance and call observe
, passing an element:
const observer = new ResizeObserver(entries => { for (let entry of entries) { // Now do something with the resized element if (entry.contentRect.width < 1000) { // Stop making AJAX calls for content... } } }); observer.observe(document.querySelector('div'));
An entry
provides you a target element as well as its dimensions and positioning:
entry = { target: div, // The element passed to `observe` contentRect: { bottom: 88, height: 88, left: 0, right: 1043, top: 0, width: 1043, x: 0, y: 0 } }
Media queries and matchMedia
provide an opportunity to adjust display via CSS but not functionality, which is where ResizeObserver
fits in.
Years ago I created a hack for spying on elements using CSS, media queries, and :before
, but it required polling via JavaScript to work properly. Having a legit, optimized JavaScript API to accomplish the same is refreshing and desperately needed!